How I Started Programming
A brief history of my coding endeavors.
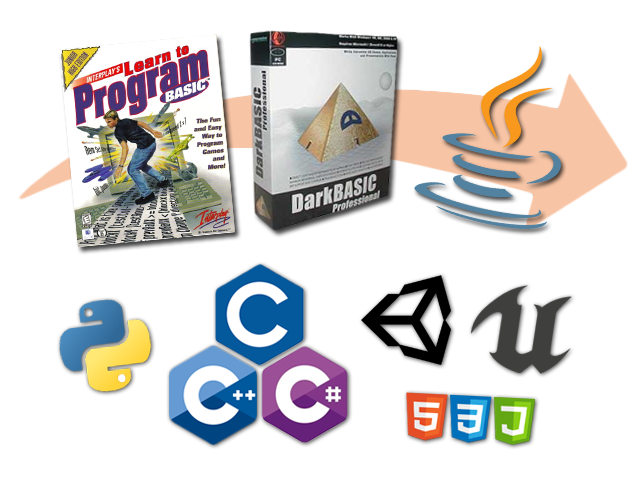
Not Pictured: Assembly, Haskell, Lua, Clojure, and others
Overview
The image for this post pretty much sums up the order of things. I started really young, programming text-based and 2D games, and I essentially just kept seeking better languages. You can skip the rest of this section if you don’t care about my life story, but I felt like writing it so here it is:
Learn to Program BASIC
When I was really young (2nd grade?) my older brother brought home Learn to Program BASIC from Interplay, which he had bought from the middle school book fair. The program featured a number of video tutorials that were over-the-top whacky to the point of being too ridiculous to use these days. Nonetheless, it was effective and it ended up teaching me to program pretty quickly.
Dark BASIC Pro
At some point (I’m going to guess 4th grade), I switched to DarkBASIC Pro from TheGameCreators. At the time I saw this as a huge upgrade because DarkBASIC did not have 100 line source limit and it came with 3D graphics and a list of features that pretty much blew my mind.
DarkBASIC was my one and only pass-time for many years. I became a certified lurker on TheGameCreators forums, where I downloaded cool games other people had made. Most of the time I read their source code for tips and tutorials. Sometimes I edited the games myself. I learned so much about 2D and 3D graphics, video game math, and programming from the members of that community.
Unfortunately, DarkBASIC is sort of dead now. They’ve released the editor and libraries completely open source on github, but even when I do manage to compile my old programs, they don’t run with any sort of speed on modern versions of Windows.
Java
My next big upgrade was Java. The same older brother that brought me Learn to Program BASIC ended up teaching me Java. He and I stayed at our grandparents’ house for a few weeks, during which I struggled with this new concept of object-oriented programming. Coming from DarkBASIC - where you start writing code at the top of your source file and it all just runs - I was frustrated that everything had to be in classes and methods. I hated having to memorize public static void main(String[] args) {}
. I met Java with some resistance but what kept me going was that it was blazing fast (yep). Compared to Dark BASIC the same sort of programs in Java would run more than 10 times as quickly.
After switching almost entirely to Java by the end of my sophomore year of high school, I missed having access to 3D graphics. I spent most of my junior year exploring OpenGL through a Java library called LWJGL. While I did have fun learning the deepest darkest secrets of 3D programming, I will definitely admit that anyone interested in 3D should just go to Unity or Unreal engine.
University
It wasn’t until I got to college that I took a real programming class. Going into college I chose Computer Engineering over Software Engineering because I was afraid I would be pretty bored with programming classes. Obviously Computer Engineering had programming too, but it was mostly Operating Systems programming, which was more about Unix than it was about learning to code. I picked up on some new languages, data-structures, and algorithms and my code quality improved a lot, though I attribute that last one to my hobbies, not my classes.
Advice
In making this post I felt I should try to share some kind of wisdom about my experience with learning to code. The thing is, I’ve tried teaching people to program before and I’m not very good at it. If I had one piece of advice for learning to code it would be this:
If you want to learn programming, you need to have passion for it.
I’m not saying you can’t learn if you aren’t in love with programming, but pick something to program that you really enjoy. Don’t let your motivation be to get a job or because programming pays well, unless those things are very motivational to you.
Game Programming
The thing that kept me interested in coding was games development and I honestly recommend game programming to everybody. It’s visual and exciting, fairly simple to code, and you can just keep adding features incrementally. It ends up being kind of exciting when you run your program and whatever you just added is there, visually. It’s even more exciting when your friends or family play your games and like them. A lot goes into game programming; You can focus on whichever aspect of the game interests you the most: AI, sound/music, 2d/pixel art, 3D modelling, graphical effects, game design, level design, etc. It helps a lot for motivation if you like playing the things you make.
Tutorials
If you want to get into game programming, I suggest you learn javascript. There are lots of tutorials online. Here are my recommended steps:
-
If you’re really new, check out Khan Academy. They’ll teach you programming basics that apply to all programming languages. You can also check out Code-cademy, though they want your money and I haven’t personally tried out their classes. They have courses aimed at various languages including Javascript.
-
Next, Download Processing. It lets you develop games and simulations (or whatever you want) using a variety of languages (including javascript) and it keeps things nice and simple.
-
Now, direct all of your love and attention at Daniel Shiffman. He’s very high energy. If that’s a problem for you, get over it; His content is thorough and informative and I personally think it’s entertaining too.
-
That’s pretty much it. You can choose to learn from whatever resources you find online. Processing itself has some pretty good documentation if you’re stuck. Learn by examples as much as possible. Find code, play with it, make modifications, and absorb its knowledge.
Code Style
One last note: there is something called “code style” and it is extremely important. You need to keep formatting in mind when writing good code. The most important thing to remember is that not everyone reading your code will have the slightest idea what you were thinking when you wrote it. That person could even be you, when you come back to an old project with no comments and bad organization and wonder “Wow, what was I doing?”.
To prevent confusion for yourself and others, I strongly recommend you learn how to comment your code properly. Code comments describe a little bit about what you’re doing when your code is less than obvious about it. They let you make notes to yourself and others.
If you can manage it, it’s also a good idea to keep your code well organized and use descriptive names for variables, classes, and methods. Make sure your methods and classes are specific and deal with only one concept and try your absolute hardest not to repeat yourself (if you’re writing the same code more than once or copy-pasting, there is probably a way to create a new method and re-use code).
Really well organized code is self-documenting, meaning that it is easier to understand and needs a lot fewer comments. This is good because fewer comments means shorter code, and shorter code is easier to read. That said, you should still comment anything that is not perfectly clear just by reading the code. Keep in mind that ‘perfectly clear’ may vary from person to person.
Finally, be consistent with you code formatting and just be aware that code style exists. There are style guides for different languages/organizations (e.g. the Google Java Style Guide). I’ve seen a lot of code while working as a TA for programming classes that would be a lot easier to understand and debug if it was properly formatted. Here are some examples of what I mean:
Capitalization
Every language has recommended capitalization for different types of identifiers (things the programmer gets to name). Make sure you’re using the right capitalization. These are the basic types:
- UpperCamelCase
- lowerCamelCase
- underscores_separating_words
- FULL_CAPS
These capitalizations apply to different things in different languages. In Java - for example - lowerCamelCase is used for variables and methods, UpperCamelCase is used for class names, FULL_CAPS is used for constants. These are not required for Java to compile your code, but it is very important to follow the standards.
Indentation
This is a big one. Almost every language uses indentation rules to make code more readable. Python actually requires specific indentaion. Here’s a C-like example:
↓ this brace is sometimes placed on the next line
if (whatever condition) {
// the stuff in here absolutely must be indented
// and it should all be indented by the same amount
}
↑ this brace is the same indentation as the start of the if statement
Whitespace
Lastly, where and how you use whitespace is important. Sometimes the style guide will tell you how to use whitespace, such as when to use new-lines, how to indent things, and whether or not to put spaces between operators/comma-separated items. The general idea behind good use of whitespace is to make your code easier to look at and hopefully easier to understand. Here is an example:
void exampleMethod() {
if (conditions) {
// some stuff
// more stuff
}
// logically similar lines of code:
StringBuilder sb = new StringBuilder();
sb.append("Hello").append(' ').append("World").append('!');
System.out.println(sb.toString());
}
In this example I am showing my preferred style, which is to put empty lines at the start and end of a method body. I keep the opening curly brace ({) at the end of almost every code line that opens it because I think it looks nicer. I also group logically related statements together and put whitespace between those logical groupings